An example of basic ajax with jquery and error handling
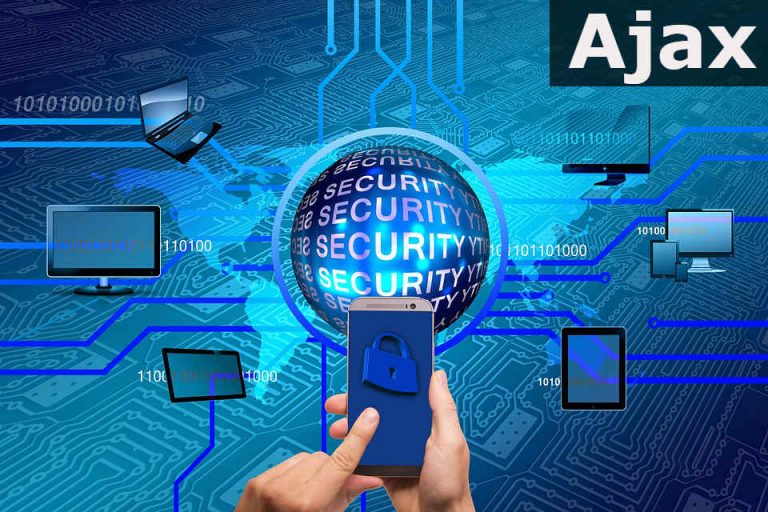
Ajax stands for Asynchronous JavaScript and XML, means using JavaScript or Jquery we can send request to server without page reload.
we can perform ajax request using JavaScript as well as Jquery, As JQuery requires less code so I always prefer Jquery over JavaScript. in this tutorial I will do it with JQuery.
lets take an example, we have 2 dropdowns 1st one is country with id=”drpCountries” and 2nd one is city with id=”drpCities”, we populate countries using php while want to fetch cities from database when we change the country at runtime.
our HTML will look like the following snippet
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 |
<!DOCTYPE html> <html> <head> <title>ALAM | Ajax example</title> </head> <script type="text/jaxascript" src="https://code.jquery.com/ui/1.11.4/jquery-ui.min.js"></script> <script type="text/jaxascript"> $(function(){ $('#drpCountries').change(function(){ var selected_country_id = $(this).val(); $.ajax({ url:'get_cities.php=', type:'POST', data:{country_id:selected_country_id}, beforeSend:function(){ $('#drpCities').html('<option value>Processing....</option>'); },success:function(response){ var obj = JSON.parse(response); $('#drpCities').html('<option value>--Select City--</option>'); $.each(obj,function(city_id, city_name){ $('#drpCities').append('<option value="'+city_id+'">'+city_name+'</option>'); }); },error:function(jqXHR, exception){ var msg = ''; if (jqXHR.status === 0) { msg = 'Not connected.\n Verify Network.'; } else if (jqXHR.status == 403) { msg = 'You are logged out, Please get login first.'; } else if (jqXHR.status == 404) { msg = 'Requested page not found. [404]'; } else if (jqXHR.status == 500) { msg = 'Internal Server Error [500].'; } else if (exception === 'parsererror') { msg = 'Requested JSON parse failed.'; } else if (exception === 'timeout') { msg = 'Time out error.'; } else if (exception === 'abort') { msg = 'Ajax request aborted.'; } else { msg = 'Uncaught Error.\n' + jqXHR.responseText; } alert(msg); }//end error });//end ajax });//end #drpCountries.click });//end $ready </script> <body> <form> <label>Countries</label> <select id="drpCountries"> <option value="1">Pakistan</option> <option value="2">USA</option> <option value="3">UAE</option> <option value="4">Oman</option> <option value="5">Bahrain</option> </select> <br> <label>Cities</label> <select id="drpCities"> <!--here we will be displaying cities via ajax--> </select> <br> <input type="submit" value="save" /> </form> </body> </html> |
for above script our php code will look like something below
1 2 3 4 5 6 7 8 9 10 11 12 |
<?php //create database connection or search mysqli to learn $sql = "select * from cities where country_id=".$_POST['country_id']; $res = $db->query($sql); $cities= array(); while($row = $res->fetch_array()){ $cities[$row['id']]=$row['city_name']; } echo json_encode($cities); ?> |
You can customize it according to your requirements, I kept it more generic and simple for beginners to understand easily. comment your issues and problems and I encourage you also to reply i you can help someone else.
Comments